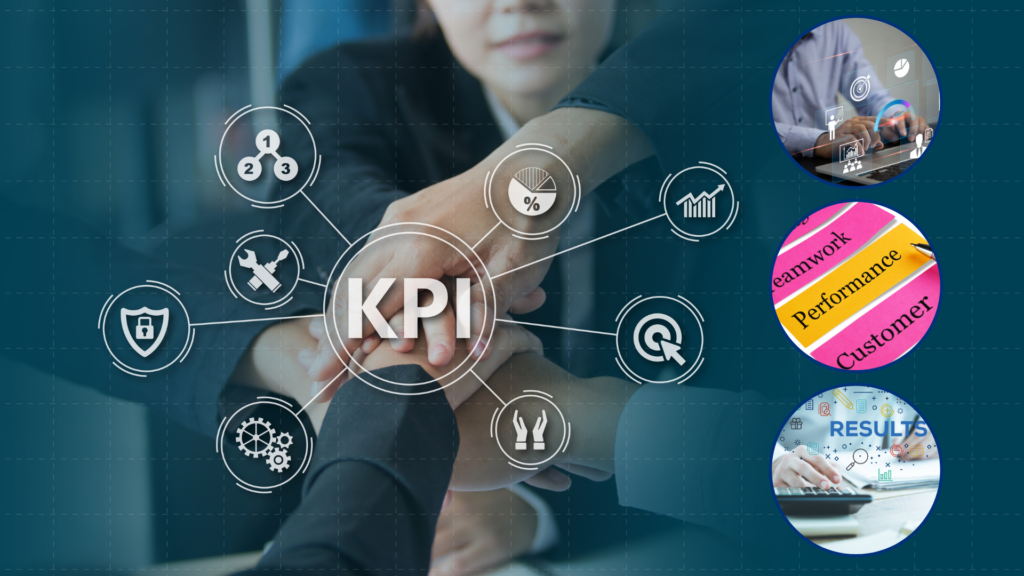
How to Get Better Performance Out of Your React Native App
In the fast-paced world of mobile app development, delivering a high-performance user experience is paramount to success. React Native, a popular framework for building cross-platform mobile apps, offers great advantages in terms of development speed and code reusability. However, ensuring optimal performance remains a critical challenge for developers.
If you’re looking to elevate your React Native app’s performance and deliver a seamless user experience, this blog post is for you. We’ll explore essential strategies and best practices that can help you achieve better performance and responsiveness, making your app stand out in the competitive app market.
Let’s dive in!
Improving performance is crucial for delivering a smooth and responsive user experience in your React Native app. Here are some effective strategies to get better performance out of your React Native app:
1. Optimize Rendering and Virtual DOM:
Use React Native’s built-in “shouldComponentUpdate” or “React.memo” to prevent unnecessary re-renders of components.
Implement “VirtualizedList” or “FlatList” for efficiently rendering large lists and grids.
Use “window.performance.mark” and “window.performance.measure” to measure performance bottlenecks during rendering.
2. Minimize UI Thread Blocking:
Offload heavy computations and operations to separate threads using Web Workers or “react-native-workers” to avoid blocking the UI thread.
Implement lazy loading and image optimization techniques to improve initial loading speed.
3. Optimize Image Loading:
Use compressed and properly sized images to reduce loading times and improve app performance.
Consider using lazy loading or progressive image loading to display images as they are downloaded.
4. Code Splitting and Dynamic Imports:
Utilize code splitting and dynamic imports to load only the necessary components and libraries when needed, reducing the initial bundle size.
Leverage “react-loadable” or “react.lazy” with “Suspense” to implement code splitting in React Native.
5. Memory Management and Garbage Collection:
Monitor and optimize memory usage to prevent memory leaks and improve app stability.
Use the “Memory” and “CPU” tabs in React Native Debugger to identify memory issues and optimize garbage collection.
6. Bundle Size Optimization:
Remove unused dependencies and code to reduce the app’s bundle size.
Implement tree shaking and dead code elimination to eliminate unused modules.
7. Use Native Modules and Libraries Wisely:
When performance is critical, consider using native modules or third-party native libraries for CPU-intensive tasks.
Avoid excessive reliance on JavaScript bridges to communicate with native code.
8. Profiling and Performance Monitoring:
Regularly profile your app using tools like “React Native Performance Monitor” or “Reactotron” to identify performance bottlenecks.
Set up crash reporting and performance monitoring tools like Firebase Crashlytics or Sentry to track and address app crashes and performance issues.
By implementing these performance optimization techniques, you can significantly enhance the speed, responsiveness, and overall user experience of your React Native app.
Remember to test your app on different devices and measure performance improvements to ensure effectiveness.
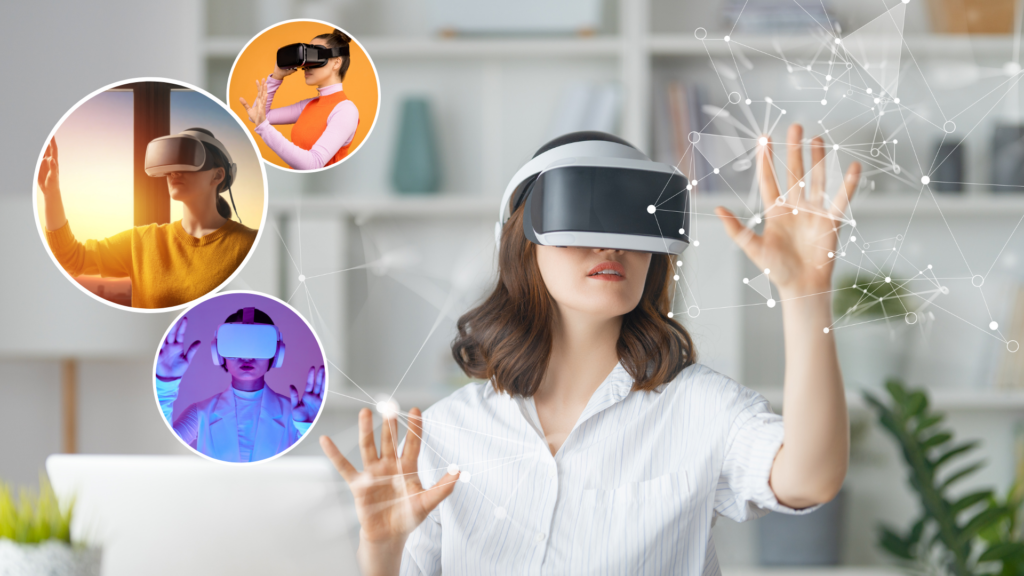
Optimizing rendering and the virtual DOM
This is a crucial aspect of improving the performance of your React Native app. Here are some specific strategies to achieve this:
1. Use shouldComponentUpdate or React.memo:
Implement the “shouldComponentUpdate” lifecycle method in class components or use “React.memo” for functional components. These help prevent unnecessary re-renders by allowing you to define conditions under which a component should update.
2. Use FlatList or VirtualizedList for Long Lists:
When dealing with large lists of data, use “FlatList” or “VirtualizedList” components instead of traditional “ScrollView” to efficiently render only the visible items on the screen. This significantly reduces the memory footprint and improves rendering performance.
3. Key Prop for List Items:
When rendering lists of items, always provide a unique “key” prop to each item. This allows React Native to efficiently track and update individual list items, avoiding unnecessary re-renders.
4. Avoid Inline Functions in Render:
Avoid defining new functions within the render method, especially when passing them as props to child components. Inline functions cause the child components to re-render unnecessarily.
5. Use PureComponent or React.memo for Optimization:
For class components, consider using “PureComponent,” which automatically implements a shallow comparison for props and state to avoid re-rendering if there are no changes. For functional components, use “React.memo” with a custom comparison function.
6. Use Animated API Wisely:
Be cautious when using the Animated API for complex animations. Complex animations can consume significant CPU resources and slow down rendering. Use simpler animations whenever possible.
7. Optimize Styling:
Minimize the use of unnecessary styles and avoid inline styles. Use stylesheets to manage styles efficiently.
Prefer using “flex” layout and other performance-optimized styles over absolute positioning for better rendering performance.
8. Use FlatList’s getItemLayout:
If you know the fixed height of items in a “FlatList,” consider using the “getItemLayout” prop to improve performance by avoiding item measurement during rendering.
9. Avoid Unnecessary Nesting:
Avoid unnecessary nesting of components, as it can lead to a deep component tree and impact performance. Flatten the component hierarchy whenever possible.
10. Use React Native Performance Tools:
Utilize tools like “React Native Debugger” and “React DevTools” to analyze and optimize component rendering, update frequency, and re-renders.
By applying these optimization techniques, you can ensure that your React Native app’s rendering and virtual DOM management are as efficient as possible, resulting in improved overall performance and a better user experience.
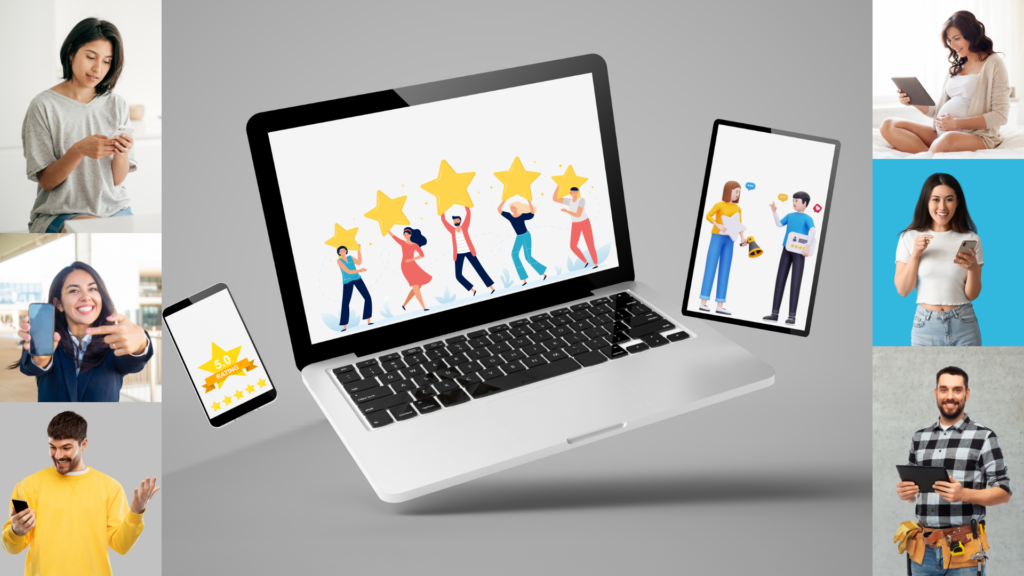
Minimizing UI thread blocking
This is essential for ensuring smooth and responsive user interfaces in your React Native app. The UI thread is responsible for rendering the user interface and responding to user interactions, so any blocking operations can lead to sluggishness and unresponsiveness. Here are some strategies to minimize UI thread blocking:
1. Use Asynchronous Operations:
Perform time-consuming tasks, such as data fetching or file I/O, in the background using asynchronous operations like Promises, async/await, or callbacks. This prevents these tasks from blocking the UI thread.
2. Avoid Lengthy JavaScript Computations:
Refrain from performing computationally intensive operations on the main thread, as they can freeze the UI. Consider breaking down complex calculations into smaller chunks and executing them asynchronously.
3. Offload Heavy Processing to Native Modules:
For tasks that are extremely resource-intensive, consider creating native modules using Java (for Android) or Objective-C/Swift (for iOS) and invoke them from your React Native app. Native modules can run on separate threads and not interfere with the UI thread.
4. Use Web Workers:
If your React Native app renders web content using WebView or other web-related components, consider using Web Workers to run JavaScript code in the background without blocking the UI.
5. Avoid Synchronous Bridge Calls:
Minimize synchronous calls between JavaScript and native code using the React Native bridge. Synchronous calls can halt the UI thread until the operation completes, leading to unresponsiveness.
6. Use Interaction Managers:
React Native provides an “InteractionManager” module that allows you to schedule tasks during idle periods, ensuring that time-sensitive UI updates take priority.
7. Chunk and Lazily Load Large Assets:
Split large assets and resources into smaller chunks and load them lazily as needed. This prevents a large initial load that could block the UI thread.
8. Optimize Animations:
Use the Animated API or third-party animation libraries to ensure that animations run smoothly without blocking the UI thread.
9. Profile and Measure Performance:
Regularly profile your app’s performance using tools like React Native Performance Monitor or Flipper to identify and resolve any performance bottlenecks related to the UI thread.
By implementing these strategies, you can minimize UI thread blocking in your React Native app, resulting in a more responsive and enjoyable user experience.
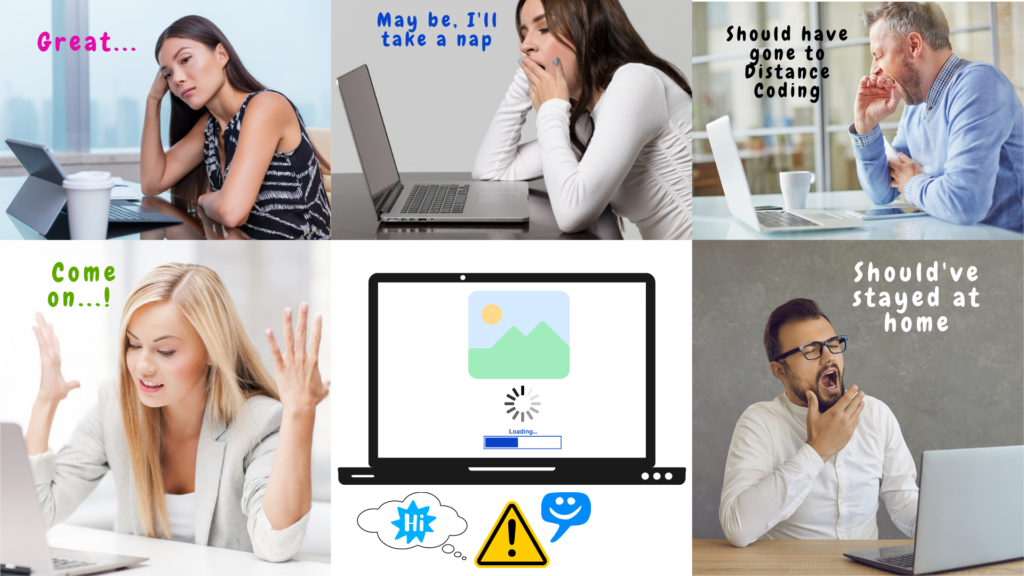
Optimizing image loading
This is crucial for improving the performance of your React Native app, as images are often a significant contributor to app size and loading times. Here are some strategies to optimize image loading in your app:
1. Image Compression:
Use image compression techniques to reduce the file size of images without compromising quality. Tools like ImageMagick, TinyPNG, or Squoosh can help you compress images before integrating them into your app.
2. Lazy Loading:
Implement lazy loading for images, especially those that are not immediately visible when the app loads. Lazy loading defers the loading of images until they are about to be displayed, reducing the initial loading time of the app.
3. Progressive Loading:
Consider using progressive image loading, where low-resolution images are displayed first and then gradually replaced with higher-resolution versions. This provides users with a better experience as images load more quickly.
4. Use React Native Image Components:
Utilize React Native’s built-in `<Image>` component, which provides performance optimizations like image caching and loading indicators. It automatically handles image loading and memory management for you.
5. Use WebP Format:
If your target platform supports it, consider using the WebP image format. WebP images offer better compression and quality compared to other formats like JPEG or PNG.
6. Platform-Specific Image Optimization:
For better performance on each platform (iOS and Android), consider using platform-specific image optimizations. For instance, Android supports WebP images natively, whereas iOS prefers the JPEG format.
7. Preload Images Strategically:
Preload important images that are likely to be displayed next or on subsequent screens. This reduces the delay when loading images on-demand and provides a smoother user experience.
8. Use Image Dimensions and Scaling:
Always specify the dimensions of images in the code to prevent layout recalculations when the images load. Also, make sure to use the appropriate scaling method based on the image’s usage (e.g., `resizeMode` prop for `<Image>` component).
9. Local vs. Remote Images:
Choose between local or remote images based on your app’s requirements. Local images load faster as they are bundled with the app, while remote images offer more flexibility for dynamic content.
10. Implement Image Caching:
Use image caching libraries like FastImage or react-native-cached-image to cache images locally. Caching allows the app to load images more quickly and reduces unnecessary network requests.
By following these image optimization techniques, you can significantly improve the performance of your React Native app, reduce loading times, and enhance the overall user experience.
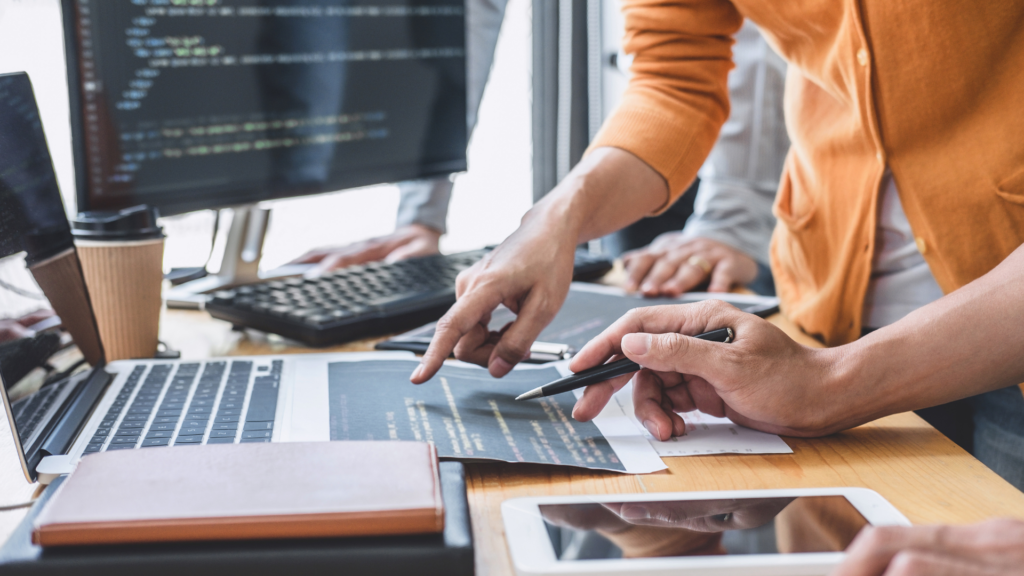
Code splitting and dynamic imports
These are powerful techniques used to optimize the performance of React Native apps by efficiently loading only the necessary code, especially for large or complex applications. They help reduce the initial bundle size and improve the app’s loading time. Let’s explore these concepts further:
1. Code Splitting:
Code splitting involves breaking the app’s codebase into smaller, more manageable chunks or “splits.” Instead of bundling the entire app into a single JavaScript file, code splitting allows you to create multiple smaller bundles that can be loaded on-demand when needed.
For example, in a React Native app, you can split code based on different screens or components. When the user navigates to a specific screen, only the code related to that screen is loaded, optimizing the initial load time and reducing the memory footprint.
2. Dynamic Imports:
Dynamic imports allow you to load JavaScript modules on-the-fly during runtime rather than during the initial bundle creation. This is achieved using dynamic import() statements.
For instance, when a user interacts with a specific feature that requires additional functionality, you can use dynamic imports to fetch and load the required modules only when necessary. This can significantly improve the app’s startup performance and overall responsiveness.
3. React.lazy() and Suspense:
React.lazy() is a built-in React function that facilitates code splitting by enabling lazy loading of components. It allows you to define a dynamic import of a component and renders a fallback UI while the component is loading.
Suspense is another React feature that works in conjunction with React.lazy(). It lets you define a loading indicator or fallback UI while the dynamically imported component is being loaded.
4. React Navigation and Code Splitting:
If you’re using React Navigation for handling app navigation, you can also take advantage of code splitting. React Navigation supports lazy loading of screen components, allowing you to load screens only when the user navigates to them, thereby optimizing the app’s performance.
Code splitting and dynamic imports are especially valuable for larger React Native apps or apps with complex features. By implementing these techniques, you can achieve faster loading times, reduce the initial bundle size, and deliver a more seamless and responsive user experience to your app’s users.
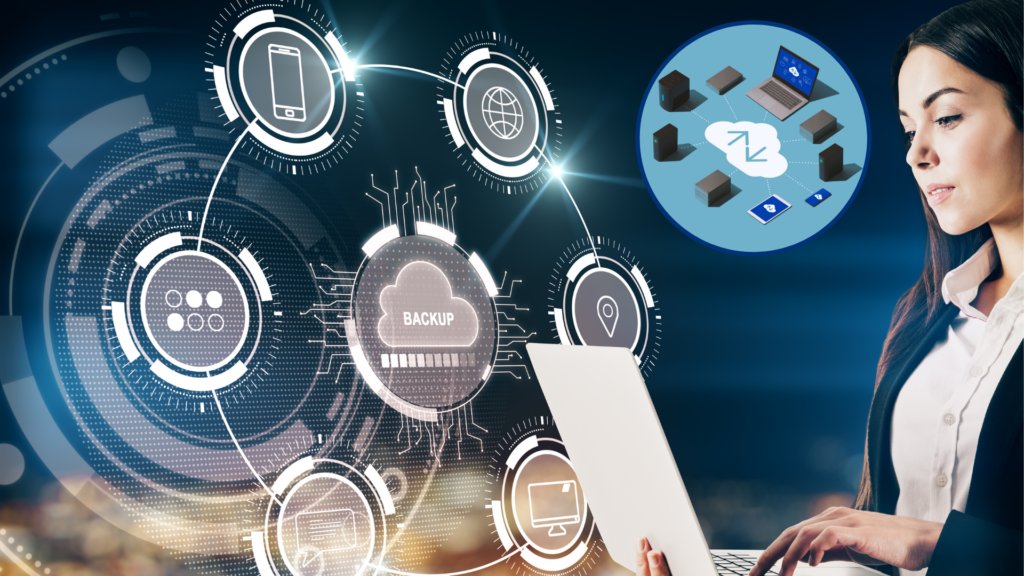
Memory management and garbage collection
These are essential concepts in any programming language, including React Native. Proper memory management ensures that your app uses memory efficiently and avoids memory leaks, which can lead to performance issues and app crashes. Here’s an overview of memory management and garbage collection in React Native:
Memory Management:
In React Native, memory management primarily revolves around managing the memory used by JavaScript and native code components. Here are some key aspects to consider:
1. JavaScript Heap:
React Native runs JavaScript code on a JavaScript engine, such as JavaScriptCore for iOS and Hermes for Android. The JavaScript heap is where objects and variables are allocated and stored during runtime. It’s crucial to write efficient and optimized code to prevent unnecessary memory consumption.
2. Native Memory:
Apart from JavaScript, React Native also interacts with native modules written in Java or Objective-C/Swift. These native modules can allocate memory on the native side, and it’s essential to ensure that the memory usage is controlled and managed efficiently.
3. Image and Asset Management:
Loading and managing images and assets can consume a significant amount of memory. To optimize memory usage, consider using compressed and scaled images and loading them dynamically as needed.
Garbage Collection:
Garbage collection is a mechanism that automatically identifies and frees up memory occupied by objects that are no longer needed by the application. JavaScript engines in React Native, such as JavaScriptCore, handle garbage collection.
1. Reference Counting:
JavaScriptCore uses reference counting as a simple form of garbage collection. When an object’s reference count reaches zero, meaning no variable or function refers to that object, it is automatically removed from memory.
2. Automatic Garbage Collection:
In addition to reference counting, modern JavaScript engines use automatic garbage collection techniques, such as Mark-and-Sweep or Generational Garbage Collection. These techniques identify and collect unreferenced objects and free up memory.
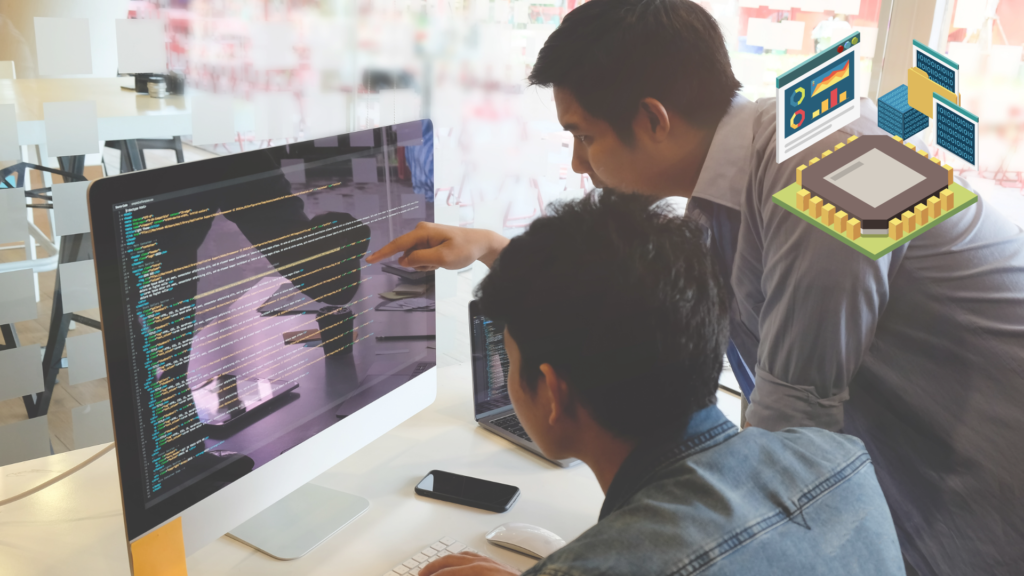
Tips for Memory Optimization
To ensure better memory management and garbage collection in your React Native app, consider the following tips:
1. Avoid creating unnecessary objects or variables that may occupy memory.
2. Use React Native’s FlatList for large lists, as it efficiently recycles views and reduces memory overhead.
3. Implement lazy loading and code splitting to load components only when needed, reducing the initial memory footprint.
4. Optimize image and asset sizes and use progressive loading techniques to reduce memory usage.
5. Test your app for memory leaks and performance bottlenecks using profiling tools.
By understanding memory management and garbage collection in React Native and applying memory optimization techniques, you can build apps that deliver a smoother user experience and perform well on a variety of devices.
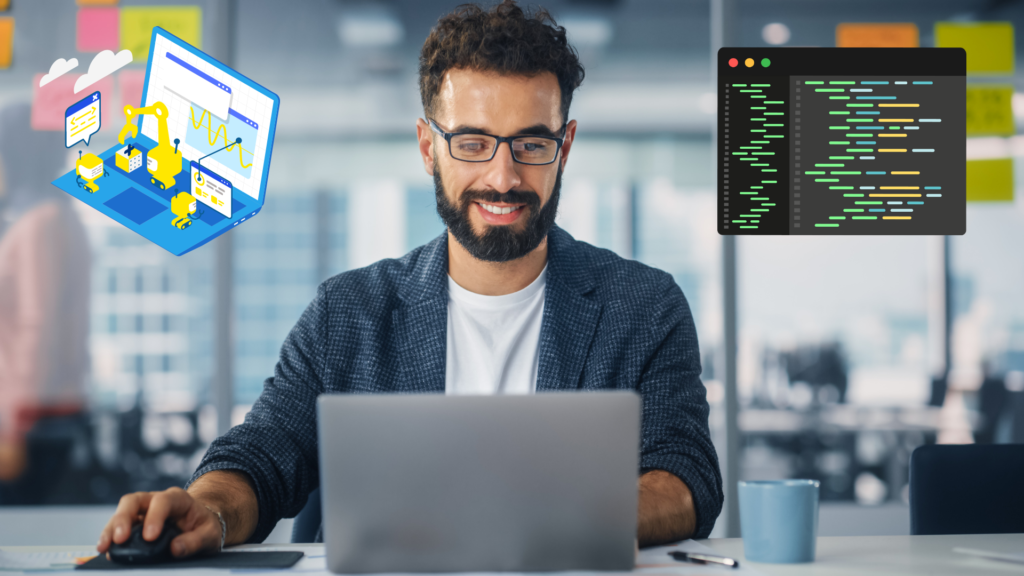
Bundle size optimization
This is a crucial aspect of improving the performance of your React Native app. It refers to reducing the size of the JavaScript bundle that is sent to the device during app installation or updates. A smaller bundle size leads to faster loading times and improved overall app performance. Here are some strategies for bundle size optimization in React Native:
1. Tree Shaking:
Tree shaking is a technique that removes unused code (dead code) from the bundle during the build process. It is achieved through static analysis of the code and identifying which parts are not being used. This eliminates unnecessary dependencies and reduces the bundle size.
2. Code Splitting:
Code splitting involves breaking down the app’s codebase into smaller chunks (or multiple bundles) instead of bundling everything into a single large file. This allows the app to load only the code that is required for the current screen or feature, reducing the initial load time.
3. Lazy Loading:
Lazy loading is a form of code splitting where certain components or modules are loaded only when they are needed, rather than during the initial app launch. This can significantly improve the app’s startup time and reduce the initial bundle size.
4. Dynamic Imports:
Dynamic imports enable loading components or modules asynchronously when requested. This is particularly useful for large libraries or features that are not required immediately. By loading them on-demand, you can keep the initial bundle size small.
5. Optimizing Images and Assets:
Compress and optimize images and other assets to reduce their size. Use tools like image compressors and SVG optimization to ensure efficient use of resources.
6. Minification:
Minification is the process of removing unnecessary characters, such as white spaces and comments, from the code to reduce its size. Tools like UglifyJS can automatically minify the JavaScript code in the bundle.
7. Avoiding Duplicate Dependencies:
Make sure you are not including duplicate dependencies in your project, as they can unnecessarily increase the bundle size. Use tools like `yarn-deduplicate` to detect and remove duplicate packages.
8. Use React Native Community Libraries:
Instead of reinventing the wheel, utilize popular and well-maintained libraries from the React Native community. These libraries are often optimized and well-tested, reducing the need to include custom code that might increase the bundle size.
9. Analyze Bundle Size:
Regularly analyze the bundle size using tools like `source-map-explorer` to identify large dependencies or sections of the code that contribute significantly to the bundle size. This will help you focus on areas that need optimization.
By following these bundle size optimization techniques, you can create a more efficient React Native app with faster loading times, better performance, and an improved user experience.
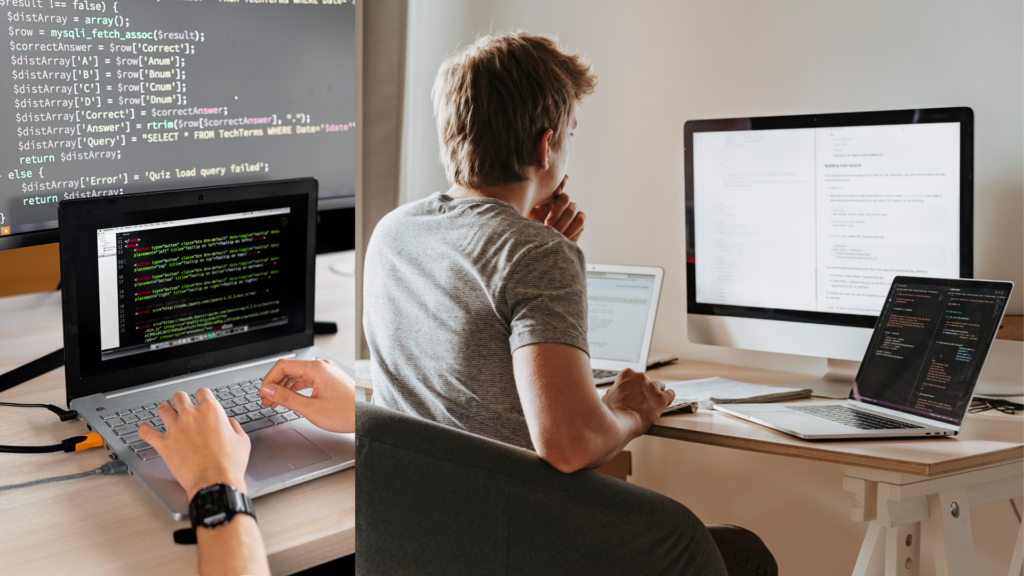
Using native modules and libraries wisely
This is another important aspect of optimizing the performance of your React Native app. Native modules allow you to access native code written in languages like Java (for Android) and Objective-C or Swift (for iOS) from your React Native JavaScript code. While native modules can provide significant performance improvements and access to device-specific functionalities, they should be used judiciously to avoid potential pitfalls. Here’s how to use native modules and libraries wisely:
1. Identify Performance-Critical Tasks:
Evaluate your app’s functionalities and identify tasks that are performance-critical and could benefit from native module implementation. For example, image processing, audio processing, or complex animations may perform better using native code.
2. Choose Reliable Native Libraries:
When using existing native libraries, choose reputable and well-maintained ones from reliable sources. Check community feedback and GitHub repositories to ensure the library is actively supported and free from major issues.
3. Avoid Unnecessary Native Calls:
Minimize the number of calls between JavaScript and native code to prevent overhead and potential performance bottlenecks. Frequent context switching between the two environments can impact app performance.
4. Implement Caching Mechanisms:
When using native modules to perform tasks like data processing or heavy calculations, consider implementing caching mechanisms to store results temporarily. This can reduce the need for repeated native calls, improving overall performance.
5. Optimize Native Code:
If you’re building custom native modules, ensure that the native code is optimized for performance. Avoid unnecessary computations and ensure memory management is handled efficiently to prevent memory leaks.
6. Use Hybrid Approach When Needed:
In some cases, a hybrid approach may be more suitable, where you combine the power of native code with JavaScript logic. For instance, complex animations can be handled natively while using React Native’s bridge to control the animation from JavaScript.
7. Keep Abstraction in Mind:
When building custom native modules, create a clear and abstracted interface to interact with the JavaScript code. This simplifies maintenance and allows for future changes without affecting other parts of the app.
8. Test Thoroughly:
Test the native modules extensively on various devices and platforms to ensure they work as expected and do not introduce any unexpected bugs or crashes.
9. Consider Alternatives:
In some cases, React Native’s JavaScript-based solutions or community-supported libraries may be sufficient to meet your performance requirements without the need for custom native modules.
By using native modules and libraries wisely, you can leverage the strengths of both React Native and native code to create a high-performance app that provides a smooth user experience on both Android and iOS devices.
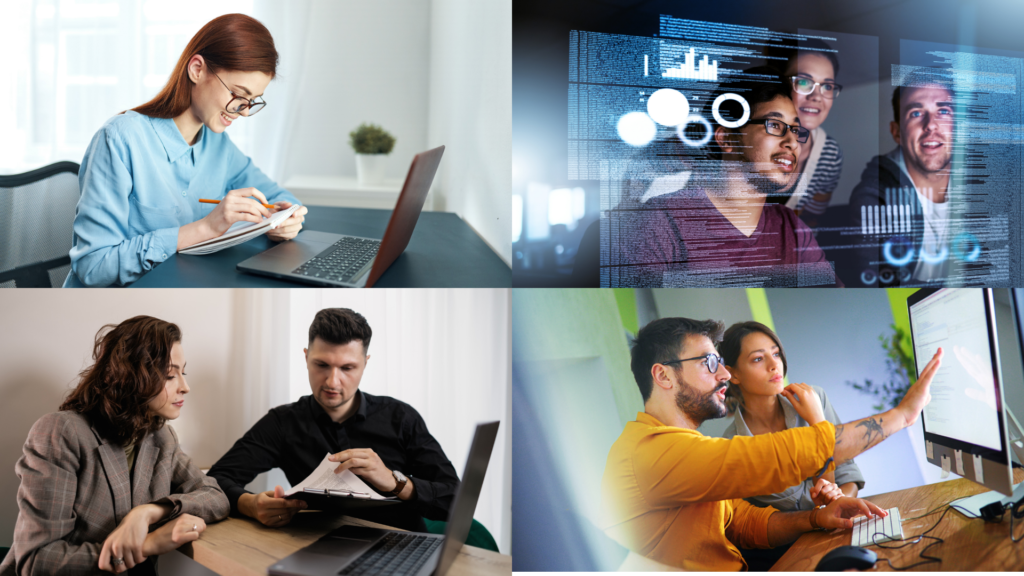
Profiling and performance monitoring
These are essential techniques to optimize the performance of your React Native app. These practices help identify performance bottlenecks, memory leaks, and areas that need improvement. By analyzing the app’s behavior and resource usage, developers can make informed decisions to enhance the overall performance. Here’s how to use profiling and performance monitoring effectively:
1. Use React Native Performance Tools:
React Native provides built-in performance tools that help you measure and analyze your app’s performance. For example, you can use the “Performance Monitor” in the React Native Developer Menu to track FPS (frames per second), CPU usage, and memory consumption in real-time.
2. Profiling with React Native Debugger:
React Native Debugger is a powerful tool that enables developers to profile and debug React Native applications. It offers advanced debugging capabilities and performance profiling tools, making it easier to pinpoint performance issues.
3. Third-Party Profiling Tools:
Consider using third-party profiling tools and performance monitoring services that offer more in-depth insights into your app’s performance. Tools like Flipper, Sentry, or New Relic can help you monitor app performance, detect crashes, and identify performance bottlenecks.
4. Analyze Rendering Performance:
Use profiling tools to analyze rendering performance and identify components that cause unnecessary re-renders. Minimizing re-renders can significantly improve app responsiveness.
5. Monitor Memory Usage:
Keep an eye on memory usage during different app interactions and user flows. Detects and fixes memory leaks to prevent crashes and improve app stability.
6. Identify Costly Operations:
Profiling tools can help you identify operations that are consuming excessive resources, such as heavy computations or inefficient data processing. Optimize or refactor these operations to reduce resource usage.
7. Analyze Network Requests:
Monitor and profile network requests to ensure they are efficient and not causing delays in app performance. Minimize unnecessary network calls and use techniques like caching to improve data loading.
8. Performance Testing on Real Devices:
Test your app’s performance on real devices, including various iOS and Android versions, to simulate real-world usage scenarios. This ensures that the app performs well on a wide range of devices.
9. Benchmarking:
Set performance benchmarks for critical app functionalities and continuously measure the app’s performance against those benchmarks. This helps you track improvements over time.
10. Regular Performance Audits:
Perform regular performance audits to keep track of any new performance issues that may arise with app updates or changes in the codebase.
By using profiling and performance monitoring, you can gain valuable insights into your app’s behavior and make data-driven decisions to optimize React Native app performance, ensuring a seamless and enjoyable user experience.
In a nutshell, optimizing the performance of your React Native app is essential for providing a top-notch user experience and gaining a competitive edge in the ever-evolving app market. By following the strategies and best practices outlined in this blog post, you can effectively enhance your app’s performance, reduce loading times, and create a smoother and more enjoyable user journey.
Remember to focus on optimizing rendering and leveraging the Virtual DOM, minimize UI thread blocking, and utilize code splitting for faster load times. Memory management, bundle size optimization, and using native modules wisely are equally crucial for enhancing your app’s efficiency.
By continuously profiling and monitoring your app’s performance, you can identify potential bottlenecks and address them promptly. Additionally, optimizing image loading and implementing caching mechanisms will further boost your app’s speed and responsiveness.
As you strive to get the most out of your React Native app, keep in mind that continuous improvement and staying updated with the latest performance-enhancing techniques will be key to staying ahead in the competitive mobile app landscape.
With these insights and techniques at your disposal, you’re now better equipped to take your React Native app to new heights of performance excellence.
Happy coding!
Contact Distance Coding https://calendly.com/distancecoding/30min today to discuss your unique needs and discover tailored solutions that align with your goals. We are here to help. Let’s talk and get to know each other. Looking forward to hearing from you.
Distance Coding – www.distancecoding.agency